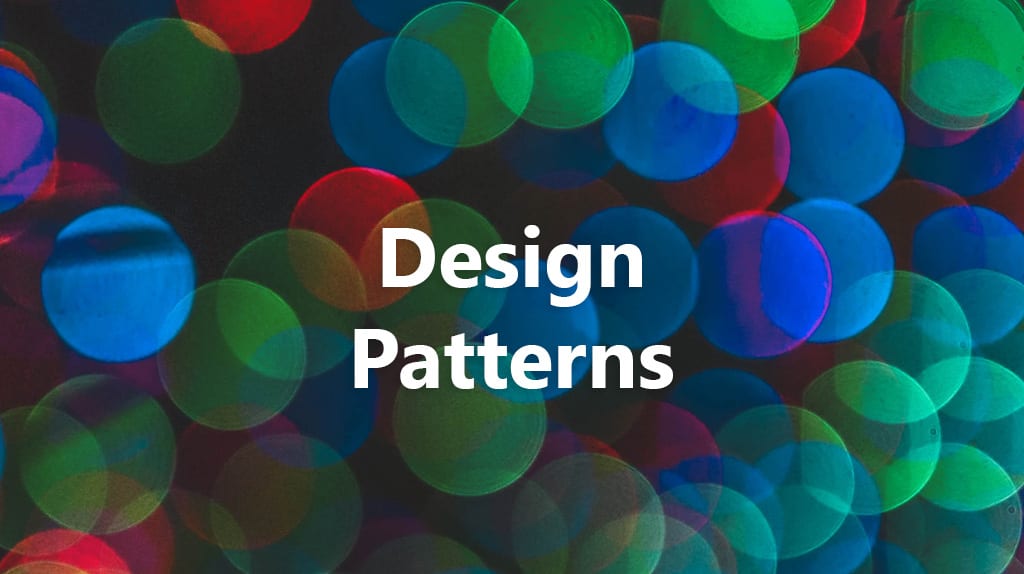
Iterator - Behavioral Design Pattern
2019, Feb 12
➿ Iterator
Provide a way to access the elements of an aggregate object sequentially without exposing its underlying representation.
interface IIterator {
public boolean hasNext();
public Object next();
}
interface IContainer {
public IIterator createIterator();
}
class Collection implements IContainer {
private String m_titles[] = {"0", "1", "2", "3", "4"};
public IIterator createIterator() {
ItemIterator result = new ItemIterator();
return result;
}
private class ItemIterator implements IIterator {
private int m_position = 0;
public boolean hasNext() {
return (m_position < m_titles.length)
}
public Object next() {
if (this.hasNext()) {
return m_titles[m_position++];
}
return null;
}
}
}