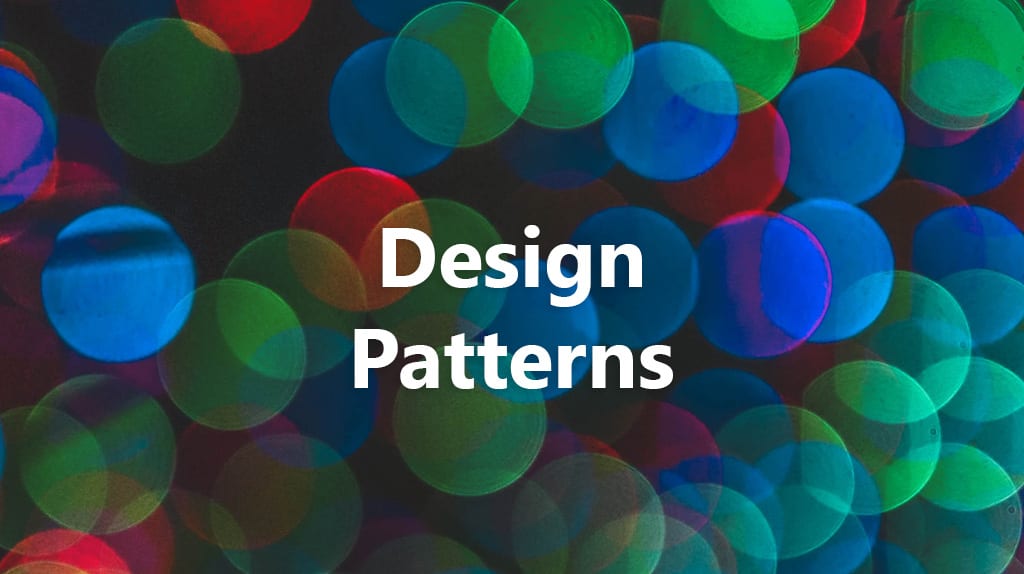
State - Behavioral Design Pattern
2019, Feb 12
🏗 State
Allow an object to alter its behavior when its internal state changes. The object will appear to change its class.
Function<String, String> upperCase = (String inputString) => inputString.toUpperCase();
Function<String, String> lowerCase = (String inputString) => inputString.toLowerCase();
Function<String, String> defaultTransform = (String inputString) => inputString;
public class TextEditor {
private Function<String, String> _transform;
TextEditor(Function<String, String> transform) {
this._transform = transform;
}
public void setTransform(Function<String, String> transform) {
this._transform = transform;
}
public void type(String words) {
System.out.println(this._transform(words));
}
}
TextEditor editor = new TextEditor(defaultTransform);
editor.type("First line");
editor.setTransform(upperCase);
editor.type("Second line");
editor.type("Third line");
editor.setTransform(lowerCase);
editor.type("Fourth line");
editor.type("Fifth line");