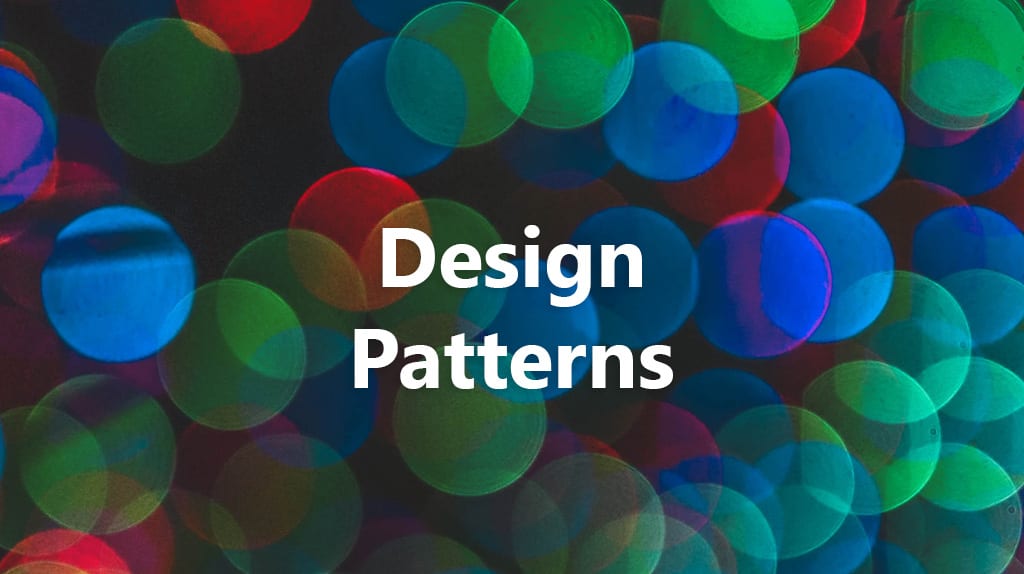
Simple Factory - Creational Design Pattern
2019, Feb 10
🏠 Simple Factory
Simple factory simply generates an instance for client without exposing any instantiation logic to the client
Example:
interface Door {
public function getWidth(): float;
public function getHeight(): float;
}
class WoodenDoor implements Door {
protected int width;
protected int height;
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public WoodenDoor(int width, int height){
this.width = width;
this.height = height;
}
}
class DoorFactory {
public static function makeDoor(width, height): Door
{
return new WoodenDoor(width, height);
}
}
When to Use? When creating an object is not just a few assignments and involves some logic, it makes sense to put it in a dedicated factory instead of repeating the same code everywhere.